SIR#
The standard Susceptible-Infected-Recovered (SIR) model features heavily throughout this documentation and so we outline its key features for anyone unfamiliar.
Warning
There is some ambiguity in the naming of the Recovered class which is also commonly referred to as Removed. In the latter sense, there is no distinction made between those who can no longer be infected due to infection acquired immunity and infection induced death. However, for more complex models, the recovered class may become susceptible again due to the effects of immune waning and deaths versus recoveries are typically important to distinguish in real world applications. Therefore, in this tutorial, the Recovered class will be reserved solely for those who survive infection.
The assumptions of the SIR model can be stated as:
An average member of the population makes contact sufficient to transmit or receive infection with \(c\) others per unit time. Each of these events carries a probability, \(p\), of transmission such that each individual has an average of \(cp = \beta\) infectious contacts per unit time. This fixed contact rate reflects what is referred to as standard incidence, as opposed to mass-action incidence, where contacts per person are proportional to the total population size, \(N\).
The population interacts heterogeneously as if a well mixed continuum. For instance, a susceptible does not have contacts with other individuals, but with the entire population on average.
The infective class recovers at a rate, \(\gamma\).
No births, deaths (natural or from disease) or migration mean there is no entry into or departure from the population: \(S(t)+I(t)+R(t)=N\).
Under these assumptions, the rates of change of the population in each compartment (Susceptible, Infected and Recovered) are given by the following equations:
We solve deterministically for flu-like parameters:
from pygom import common_models
import matplotlib.pyplot as plt
import numpy as np
import random
import math
#####################
# Set up PyGOM object
#####################
# Parameters
n_pop=1e4
gamma=1/4
R0=1.3
beta=R0*gamma
ode = common_models.SIR({'beta':beta, 'gamma':gamma, 'N':n_pop})
# Time range and increments
tmax=365 # maximum time over which to run solver
dt=0.1 # timestep
n_timestep=math.ceil(tmax/dt) # number of iterations
t = np.linspace(0, tmax, n_timestep) # times at which solution will be evaluated
# Initial conditions
i0=1
x0=[n_pop-i0, i0, 0]
ode.initial_values = (x0, t[0])
# Deterministic evolution
solution=ode.solve_determ(t[1::])
Plotting the result recovers the familiar epidemic trajectory:
Show code cell source
f, axarr = plt.subplots(1,3, layout='constrained', figsize=(10, 4))
# Plot colours
colours=["C1", "C0", "C2"]
stateList=["S", "I", "R"]
for i in range(0, 3):
axarr[i].plot(t, solution[:,i], color=colours[i])
axarr[i].set_ylabel(stateList[i], rotation=0)
axarr[i].set_xlabel('Time')
plt.show()
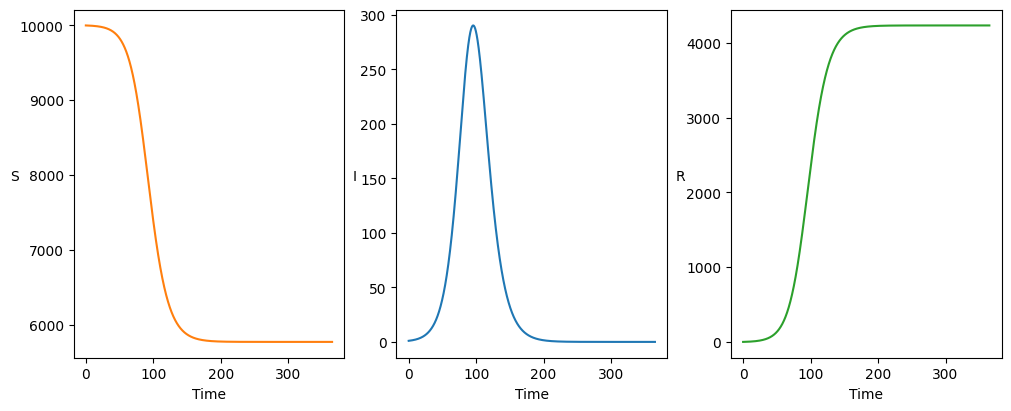